[06 Feb 2020] Check for balanced parentheses in an expression
Check for balanced parentheses in an expression
Given an expression string exp , write a program to examine whether the pairs and the orders of “{“,”}”,”(“,”)”,”[“,”]” are correct in exp.
Example:
Input: exp = “[()]{}{[()()]()}”
Output: BalancedInput: exp = “[(])”
Output: Not Balanced
Algorithm:
- Declare a character stack S.
- Now traverse the expression string exp.
- If the current character is a starting bracket (‘(‘ or ‘{‘ or ‘[‘) then push it to stack.
- If the current character is a closing bracket (‘)’ or ‘}’ or ‘]’) then pop from stack and if the popped character is the matching starting bracket then fine else parenthesis are not balanced.
- After complete traversal, if there is some starting bracket left in stack then “not balanced”
Below image is a dry run of the above approach:
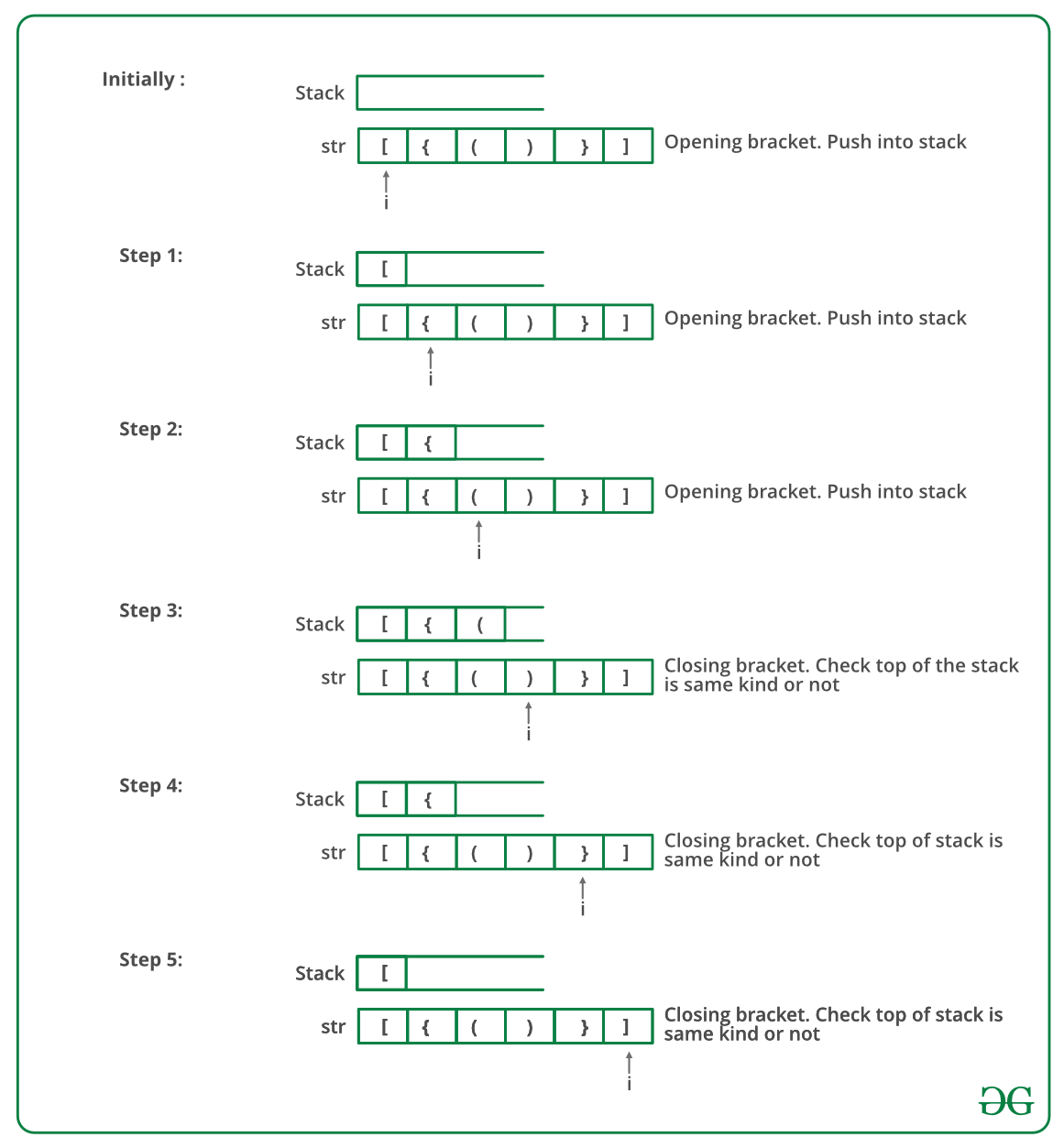
Below is the implementation of the above approach:
Output:
Balanced
Time Complexity: O(n)
Auxiliary Space: O(n) for stack.
Auxiliary Space: O(n) for stack.
Output:
Balanced
Time Complexity: O(n)
Auxiliary Space: O(n) for stack.
Auxiliary Space: O(n) for stack.
Output:
Balanced
Time Complexity: O(n)
Auxiliary Space: O(n) for stack.
Auxiliary Space: O(n) for stack.
Comments
Post a Comment